In this post, we will learn how to use AnimatedBuilder to make a Flutter blinking icon.
If you want to create blinking text, read this post first.
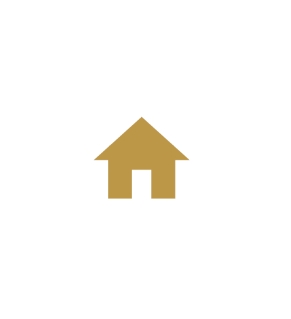
class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Home'), ), backgroundColor: Colors.white, body: SafeArea( child: Center(child: BlinkIcon()), ), ); } } class BlinkIcon extends StatefulWidget{ @override _BlinkIconState createState() => _BlinkIconState(); } class _BlinkIconState extends State<BlinkIcon> with SingleTickerProviderStateMixin{ AnimationController _controller; Animation<Color> _colorAnimation; @override void initState() { _controller = AnimationController(vsync: this, duration: Duration(milliseconds: 500)); _colorAnimation = ColorTween(begin: Colors.blue, end: Colors.orange) .animate(CurvedAnimation(parent: _controller, curve: Curves.linear)); _controller.addStatusListener((status) { if (status == AnimationStatus.completed) { _controller.reverse(); } else if (status == AnimationStatus.dismissed) { _controller.forward(); } setState(() {}); }); _controller.forward(); super.initState(); } @override Widget build(BuildContext context) { return AnimatedBuilder( animation: _controller, builder: (context, child) { return Icon(Icons.home, size: 128, color: _colorAnimation.value,); }, ); } }