AnimatedAlign can automatically transition the child’s position over a given duration whenever the given alignment changes.
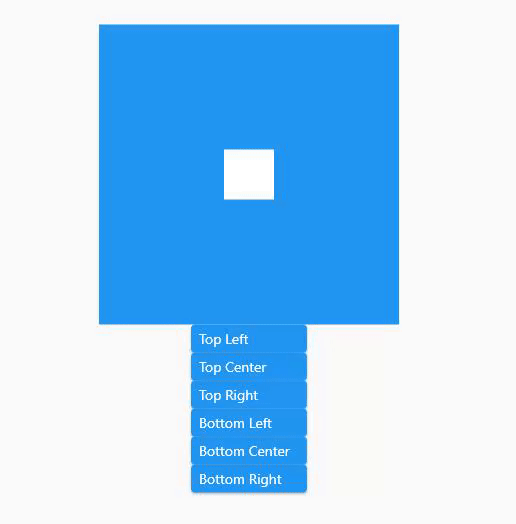
import 'package:flutter/material.dart';
class HomePage extends StatefulWidget {
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
Alignment _alignment = Alignment.center;
void _move(Alignment alignment) {
setState(() {
_alignment = alignment;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Demo'),
),
body: SafeArea(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
padding: EdgeInsets.all(16.0),
width: 300,
height: 300,
color: Colors.blue,
child: AnimatedAlign(
alignment: _alignment,
duration: Duration(seconds: 1),
child: Container(
width: 50.0,
height: 50.0,
color: Colors.white,
)),
),
ElevatedButton(
onPressed: () => _move(Alignment.topLeft),
child: Container(width: 100, child: Text('Top Left'))),
ElevatedButton(
onPressed: () => _move(Alignment.topCenter),
child: Container(width: 100, child: Text('Top Center'))),
ElevatedButton(
onPressed: () => _move(Alignment.topRight),
child: Container(width: 100, child: Text('Top Right'))),
ElevatedButton(
onPressed: () => _move(Alignment.bottomRight),
child: Container(width: 100, child: Text('Bottom Left'))),
ElevatedButton(
onPressed: () => _move(Alignment.bottomCenter),
child: Container(width: 100, child: Text('Bottom Center'))),
ElevatedButton(
onPressed: () => _move(Alignment.bottomLeft),
child: Container(width: 100, child: Text('Bottom Right'))),
],
),
),
),
);
}
}
import 'package:flutter/material.dart';
class HomePage extends StatefulWidget {
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
Alignment _alignment = Alignment.center;
void _move(Alignment alignment) {
setState(() {
_alignment = alignment;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Demo'),
),
body: SafeArea(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
padding: EdgeInsets.all(16.0),
width: 300,
height: 300,
color: Colors.blue,
child: AnimatedAlign(
alignment: _alignment,
duration: Duration(seconds: 1),
child: Container(
width: 50.0,
height: 50.0,
color: Colors.white,
)),
),
ElevatedButton(
onPressed: () => _move(Alignment.topLeft),
child: Container(width: 100, child: Text('Top Left'))),
ElevatedButton(
onPressed: () => _move(Alignment.topCenter),
child: Container(width: 100, child: Text('Top Center'))),
ElevatedButton(
onPressed: () => _move(Alignment.topRight),
child: Container(width: 100, child: Text('Top Right'))),
ElevatedButton(
onPressed: () => _move(Alignment.bottomRight),
child: Container(width: 100, child: Text('Bottom Left'))),
ElevatedButton(
onPressed: () => _move(Alignment.bottomCenter),
child: Container(width: 100, child: Text('Bottom Center'))),
ElevatedButton(
onPressed: () => _move(Alignment.bottomLeft),
child: Container(width: 100, child: Text('Bottom Right'))),
],
),
),
),
);
}
}
import 'package:flutter/material.dart'; class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { Alignment _alignment = Alignment.center; void _move(Alignment alignment) { setState(() { _alignment = alignment; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Demo'), ), body: SafeArea( child: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Container( padding: EdgeInsets.all(16.0), width: 300, height: 300, color: Colors.blue, child: AnimatedAlign( alignment: _alignment, duration: Duration(seconds: 1), child: Container( width: 50.0, height: 50.0, color: Colors.white, )), ), ElevatedButton( onPressed: () => _move(Alignment.topLeft), child: Container(width: 100, child: Text('Top Left'))), ElevatedButton( onPressed: () => _move(Alignment.topCenter), child: Container(width: 100, child: Text('Top Center'))), ElevatedButton( onPressed: () => _move(Alignment.topRight), child: Container(width: 100, child: Text('Top Right'))), ElevatedButton( onPressed: () => _move(Alignment.bottomRight), child: Container(width: 100, child: Text('Bottom Left'))), ElevatedButton( onPressed: () => _move(Alignment.bottomCenter), child: Container(width: 100, child: Text('Bottom Center'))), ElevatedButton( onPressed: () => _move(Alignment.bottomLeft), child: Container(width: 100, child: Text('Bottom Right'))), ], ), ), ), ); } }