AnimatedContainer is an animated version of Flutter Container. It gradually changes its values over a defined duration. This widget automatically animate between the old and new values of properties such as height, box decoration when they change.
Table of Contents
Bar chart animation
Each bar in the chart is an AnimatedContainer widget. The height is changeable.
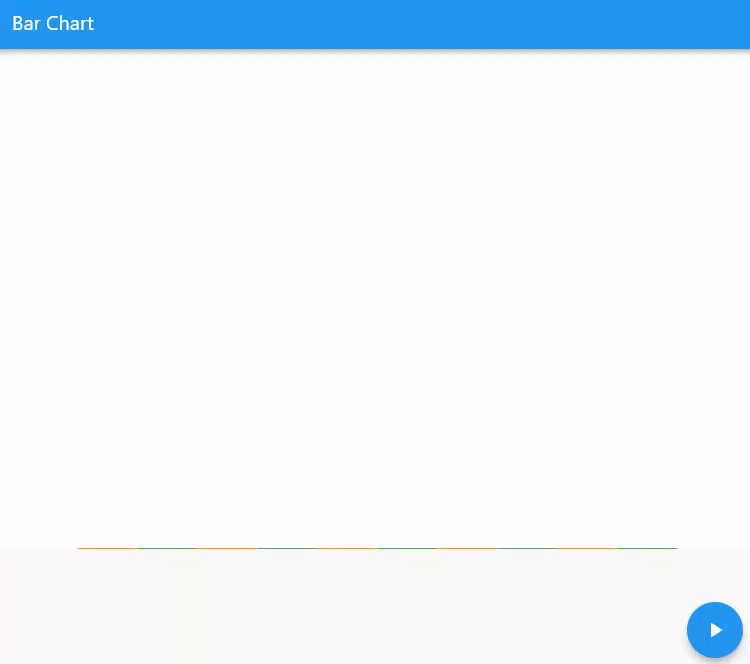
import 'dart:math'; import 'package:flutter/material.dart'; class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { List<double> heights = []; @override void initState() { super.initState(); for (var i = 0; i < 10; i++) { heights.add(1); } } void animateBarChart() { setState(() { for (var i = 0; i < 10; i++) { heights[i] = Random().nextInt(200).toDouble(); } }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Bar Chart'), ), body: SafeArea( child: Container( height: 500, color: Colors.white, child: Row( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.end, children: [ ...List.generate(10, (index) { return AnimatedContainer( padding: EdgeInsets.all(16.0), duration: Duration(seconds: 2), width: 60, height: heights[index], color: index % 2 == 0 ? Colors.orange : Colors.green, child: Text('${heights[index].toInt()}'), ); }) ], ), )), floatingActionButton: FloatingActionButton( onPressed: animateBarChart, child: Icon(Icons.play_arrow), ), ); } }
Animated BoxDecoration
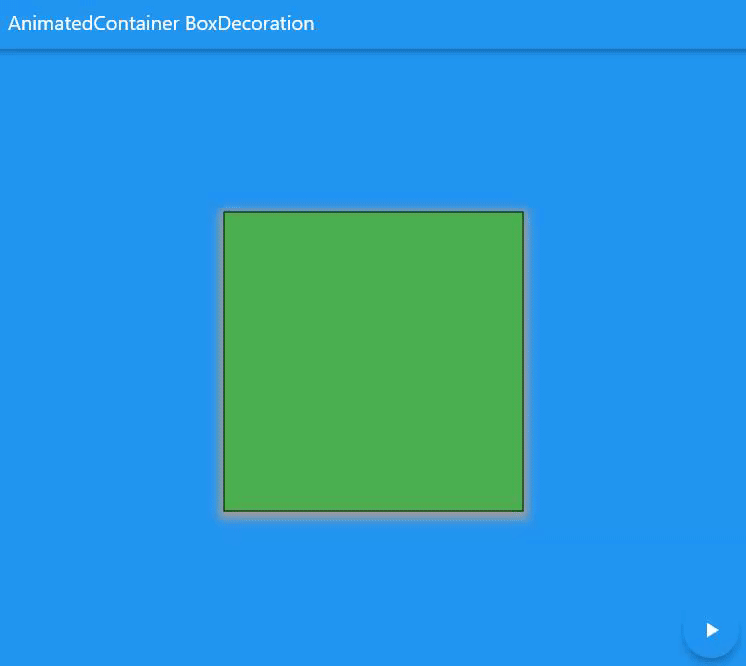
import 'dart:math'; import 'package:flutter/material.dart'; class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { bool _start = true; @override void initState() { super.initState(); } void animateBarChart() { setState(() { _start = !_start; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('AnimatedContainer BoxDecoration'), ), body: SafeArea( child: Container( color: Colors.blue, child: Center( child: AnimatedContainer( padding: EdgeInsets.all(16.0), duration: Duration(seconds: 2), width: _start ? 300 : 150, height: _start ? 300 : 150, child: Text(''), decoration: BoxDecoration( borderRadius: BorderRadius.circular(_start ? 0 : 10), border: Border( top: BorderSide(color: _start ? Colors.black : Colors.white), bottom: BorderSide(color: _start ? Colors.black : Colors.white), left: BorderSide(color: _start ? Colors.black : Colors.white), right: BorderSide(color: _start ? Colors.black : Colors.white), ), color: _start ? Colors.green : Colors.redAccent, boxShadow: _start ? [ BoxShadow( color: Colors.grey, spreadRadius: 5, blurRadius: 7, offset: Offset(0, 3), // changes position of shadow ), ] : [], ), ), ), )), floatingActionButton: FloatingActionButton( onPressed: animateBarChart, child: Icon(Icons.play_arrow), ), ); } }
You can browse more content about Flutter’s buttons here.